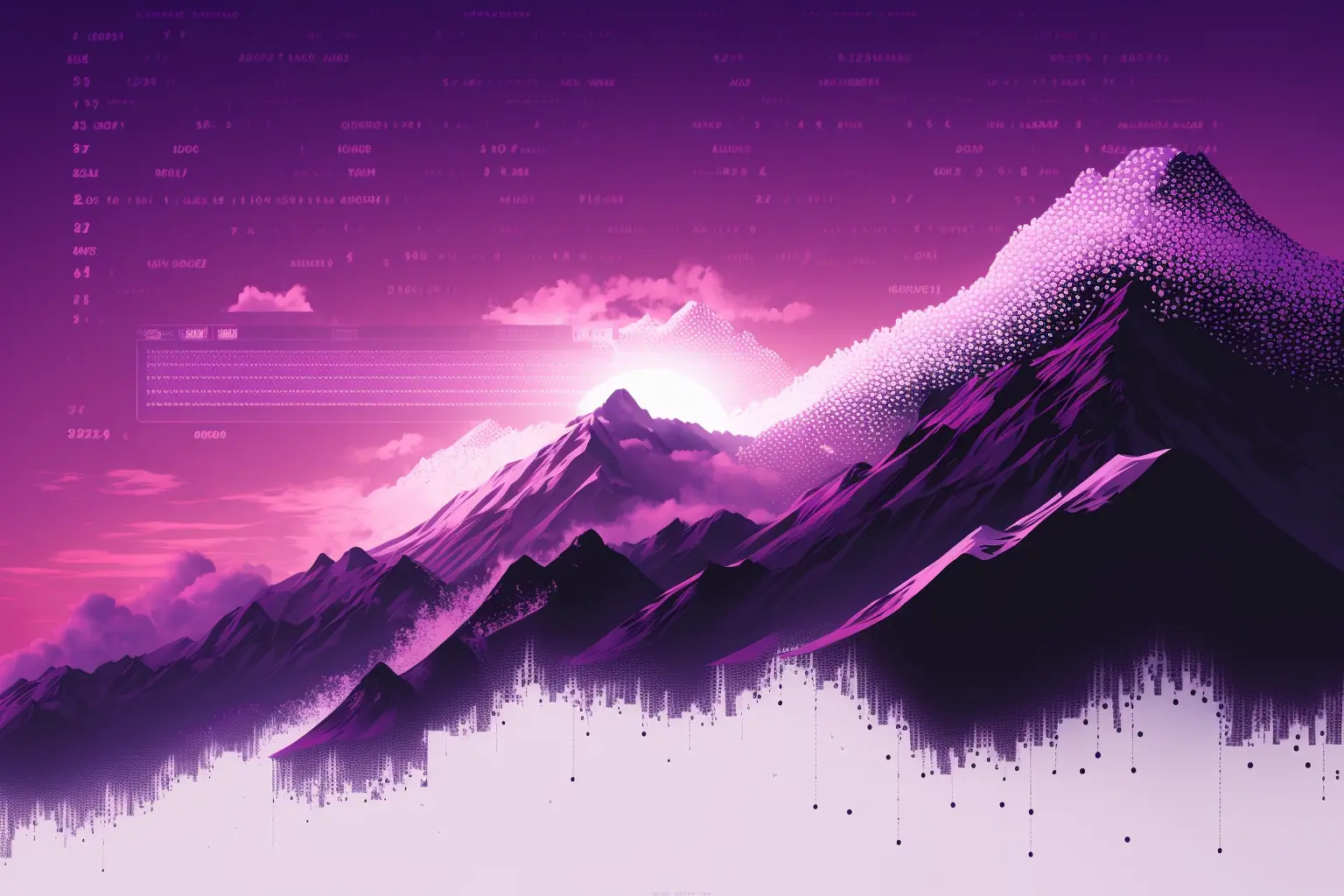
Dear Language Designers: Please copy `where` from Haskell
where` allows to extract part of your code and give it a new name, not like a local variable or a private function.
An indie hacker, a problem solver and serial project holder. I build projects to make the life of others and mine easier. This page is intended to collect all my projects and some thoughts.